DionysusToast
Legendary member
- Messages
- 5,965
- Likes
- 1,501
All
I recently had the need to hook Ninja up to Excel. After perusing various forums, I couldn't find any instructions on how to do it. In the end, I had to figure it out myself. I posted the instructions below on another, less public forum before Christmas but decided since then to give it to a wider audience.
If you want Ninja hooked up to Excel - here's how to do it...
Microsoft Interop
This is a pre-requisite. It allows communications between Ninja & Excel. Microsoft has 2 versions:
Office 2003
Download details: Office 2003 Update: Redistributable Primary Interop Assemblies
Office 2007
Download details: 2007 Microsoft Office System Update: Redistributable Primary Interop Assemblies
The particular dll we are interested from is: Interop.Microsoft.Office.Interop.Excel.dll
The target directory for this dll should be: <My Documents>\NinjaTrader 7\bin\Custom
You can either install the Interop Assemblies into this directory or just move the dll file there.
Referencing the DLL in Ninja
Next you need to create a reference to the Interop DLL in Ninja. To do this, you need to get to the references window which is available when editing an indicator. Just choose any indicator for this.
Open Ninja Control Centre -> Tools -> Edit NinjaScript -> Indicator -> Select ANY Indicator
Once the indicator is open, right click in the body of the indicator window and select "References". You will see a window appear that looks like this:
Click "Add", it will then open up an explorer window looking at the <My Documents>\NinjaTrader 7\bin\Custom directory - locate the dll and click "insert"
Then you should see the following in the references window:
Now you are ready to go and start writing code to integrate with Excel
to be continued...
I recently had the need to hook Ninja up to Excel. After perusing various forums, I couldn't find any instructions on how to do it. In the end, I had to figure it out myself. I posted the instructions below on another, less public forum before Christmas but decided since then to give it to a wider audience.
If you want Ninja hooked up to Excel - here's how to do it...
Microsoft Interop
This is a pre-requisite. It allows communications between Ninja & Excel. Microsoft has 2 versions:
Office 2003
Download details: Office 2003 Update: Redistributable Primary Interop Assemblies
Office 2007
Download details: 2007 Microsoft Office System Update: Redistributable Primary Interop Assemblies
The particular dll we are interested from is: Interop.Microsoft.Office.Interop.Excel.dll
The target directory for this dll should be: <My Documents>\NinjaTrader 7\bin\Custom
You can either install the Interop Assemblies into this directory or just move the dll file there.
Referencing the DLL in Ninja
Next you need to create a reference to the Interop DLL in Ninja. To do this, you need to get to the references window which is available when editing an indicator. Just choose any indicator for this.
Open Ninja Control Centre -> Tools -> Edit NinjaScript -> Indicator -> Select ANY Indicator
Once the indicator is open, right click in the body of the indicator window and select "References". You will see a window appear that looks like this:
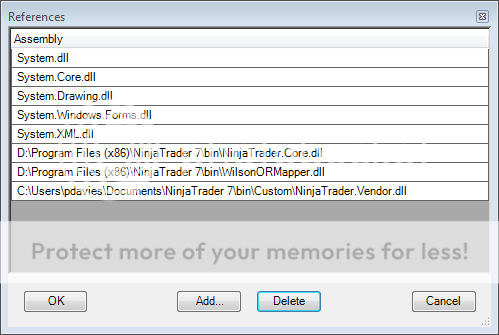
Click "Add", it will then open up an explorer window looking at the <My Documents>\NinjaTrader 7\bin\Custom directory - locate the dll and click "insert"
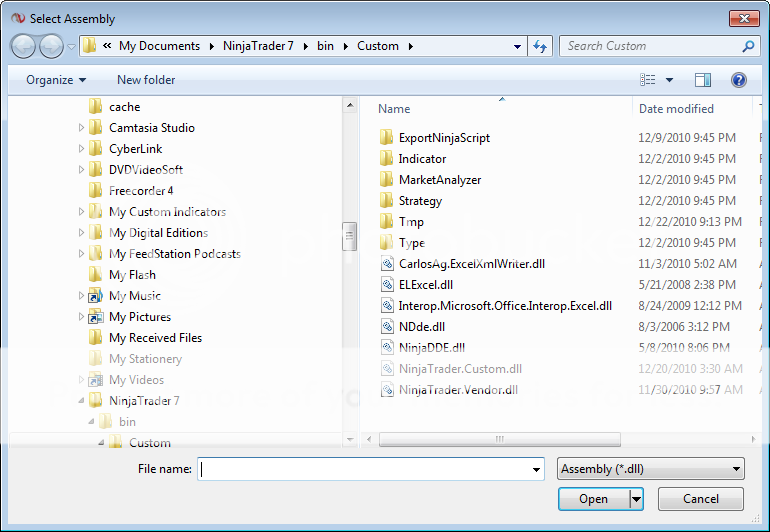
Then you should see the following in the references window:
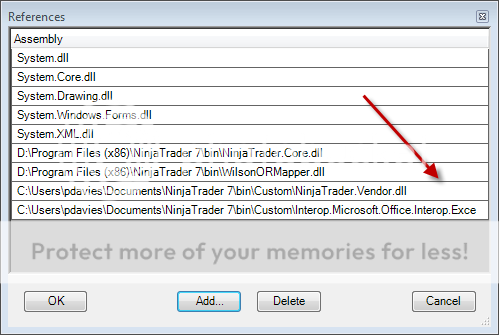
Now you are ready to go and start writing code to integrate with Excel
to be continued...
Last edited: